Javascript Generators -> Practical use case
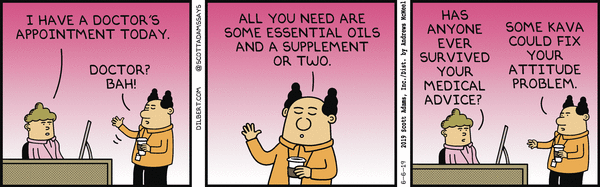
Hi Guys, it's been a while since I didn't write anything, but today I want to share with you something I faced today, while I was reviewing the code of one of my teammates did, I saw a "good" implementation of how to deal with a paginated endpoint. Basically he used recursion in order to iterate the pages, something like this: function handlePages(response) { const res = { data : response.data } if (response.pages.next_page) { res.next = async () => { const nextResponse = await request(response.pages.next_page) return handlePages(nextResponse) } } return res } It works! but I don't like the recursion part, because if we need to iterate it we need some extra logic: const response = await request(firstPageUrl) let page = handlePages(response) while (response.next) { // Complex logic here response = await response.next() } So far, this logic looks great, bu